I haven’t written on listboxes in a long while, and since I’ve recently posted on related controls (editbox, combo-box), I thought of following up with an article on customizing Matlab listbox layout. By the end of today’s article, you should be able to customize the layout of items within your listbox, such as the following:

Customized listbox layouts
For the following hacks, we need to gain access to the listbox control’s underlying Java component, which is a MathWorks class that extends the standard JList. We can get this component’s reference using the findjobj utility:
>> hListbox = uicontrol('Style','List', 'String',{'item #1','item #2'}); >> jScrollPane = java(findjobj(hListbox)) jScrollPane = com.mathworks.hg.peer.utils.UIScrollPane[...] >> jListbox = jScrollPane.getViewport.getView jListbox = com.mathworks.hg.peer.ListboxPeer$UicontrolList[...]
Like multi-line editboxes, listboxes are actually composed of a container (a com.mathworks.hg.peer.utils.UIScrollPane
object) that includes three children, as expected from any JScrollPane: a javax.swing.JViewport
that contains the ListboxPeer$UicontrolList
component, and horizontal/vertical scrollbars. I explained how to customize the scrollbars in an article back in early 2010.
Today we are not interested in the scroll-pane or scollbars, but rather the jListbox
component that takes up the view-port’s contents. This component includes many useful properties that we can access and modify, including several that control the layout of the list items:
LayoutOrientation sets the layout of listbox items within the viewport. Possible values include:
- The default value (
jListbox.VERTICAL
=0) indicates the regular top-to-bottom arrangement jListbox.VERTICAL_WRAP
=1 sets a horizontal item layout, wrapping to a new row as necessary for the maximum number of rows determined by the VisibleRowCount property (default=8)jListbox.HORIZONTAL_WRAP
=2 sets a vertical item layout, wrapping to a new column at row number VisibleRowCount
For example:
jListbox.setLayoutOrientation(jListbox.HORIZONTAL_WRAP); jListbox.setVisibleRowCount(3); set(jListbox, 'LayoutOrientation',2, 'VisibleRowCount',3); % equivalent alternative
![]() LayoutOrientation = VERTICAL = 0VisibleRowCount is irrelevant | ![]() LayoutOrientation = VERTICAL_WRAP = 1VisibleRowCount = 3 | ![]() LayoutOrientation = HORIZONTAL_WRAP = 2VisibleRowCount = 3 |
FixedCellHeight and FixedCellWidth hold the listbox’s cells height (default=13 pixels) and width (default=-1). A value of -1 means that the actual size is determined by the default platform-dependent CellRenderer size:
![]() FixedCellHeight = -1 FixedCellWidth = -1 | ![]() FixedCellHeight = 10 FixedCellWidth = 30 | ![]() FixedCellHeight = 16 FixedCellWidth = 50 |
We can use these properties to display a selection matrix of icons. For example, let’s display the icons in Matlab standard icons folder:
% Prepare the list of ImageIcon objects iconsFolder = fullfile(matlabroot,'toolbox/matlab/icons'); imgs = dir(fullfile(iconsFolder,'*.gif')); for iconIdx = 1 : length(imgs) iconFilename = fullfile(iconsFolder,imgs(iconIdx).name); iconFilename = strrep(iconFilename, '\', '/'); htmlStr{iconIdx} = ['<html><img src="file:/' iconFilename '"/>']; % no need for </html> end % Display in a standard one-column listbox hListbox = uicontrol('style','list', 'string',htmlStr, 'position',[10,10,160,90]); % Modify the listbox layout to display 18x18-pixel cells jScrollPane = findjobj(hListbox); jListbox = jScrollPane.getViewport.getView; jListbox.setLayoutOrientation(jListbox.HORIZONTAL_WRAP) jListbox.setVisibleRowCount(4) jListbox.setFixedCellWidth(18) % icon width=16 + 2px margin jListbox.setFixedCellHeight(18) % icon height=16 + 2px margin jListbox.repaint % refresh the display

Customized listbox layout
Other interesting things that we can do with listboxes (among others):
- Customize the scrollbars, as noted above
- Display HTML-formatted list items:
uicontrol('Style','list', 'Position',[10,10,70,70], 'String', ... {'<html><font color="red">Hello</font></html>', 'world', ... '<html><font style="font-family:impact;color:green"><i>What a', ... % note: </i></font></html> are not needed '<html><font color="blue" face="Comic Sans MS">nice day!</font>'}); % note: </html> is not needed
Listbox with HTML colored items
- Setting dynamic tooltips and right-click context-menus:
Listbox dynamic tooltip
Listbox dynamic context (right-click) menu
Note that while the code above used the underlying Java component, absolutely no knowledge of Java is required to understand it or use it. In fact, the entire code above is pure Matlab, simply setting the component’s properties and calling its methods, and using its inherent support of HTML strings.
Much more advanced customizations are possible at the Java level, especially using a dedicated CellRenderer. Interested readers can find more information about these and other possible customizations in my report on “Advanced Customizations of Matlab Uicontrols“. This 90-page PDF report can be purchased here ($39, please allow 24 hours for delivery by email). The report explains how to customize Matlab’s uicontrols in ways that are simply not possible using documented Matlab properties. This includes treatment of push buttons, toggle buttons, radio buttons, checkboxes, editboxes, listboxes, popup menus (aka combo-boxes/drop-downs), sliders, labels, and tooltips. Much of the information in the report is also available in hard-copy format in chapter 6 of my Matlab-Java programming book.
If you’d like me to personally add similar magic to your GUI, email me to see if I can help.
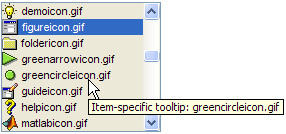
Advanced listbox CellRenderer customization
Now tell the truth – doesn’t Matlab’s standard listbox look kinda boring after all this?
Related posts:
- Setting listbox mouse actions Matlab listbox uicontrol can be modified to detect mouse events for right-click context menus, dynamic tooltips etc....
- GUIDE customization Matlab's GUI Design Editor (GUIDE) has several interesting undocumented features. This post describes how to customize GUIDE rulers....
- Button customization Matlab's button uicontrols (pushbutton and togglebutton) are basically wrappers for a Java Swing JButton object. This post describes how the buttons can be customized using this Java object in ways...
- Uitable customization report In last week’s report about uitable sorting, I offered a report that I have written which covers uitable customization in detail. Several people have asked for more details about the...